API Reference
Interface any scripts with TofuPilot's REST API.
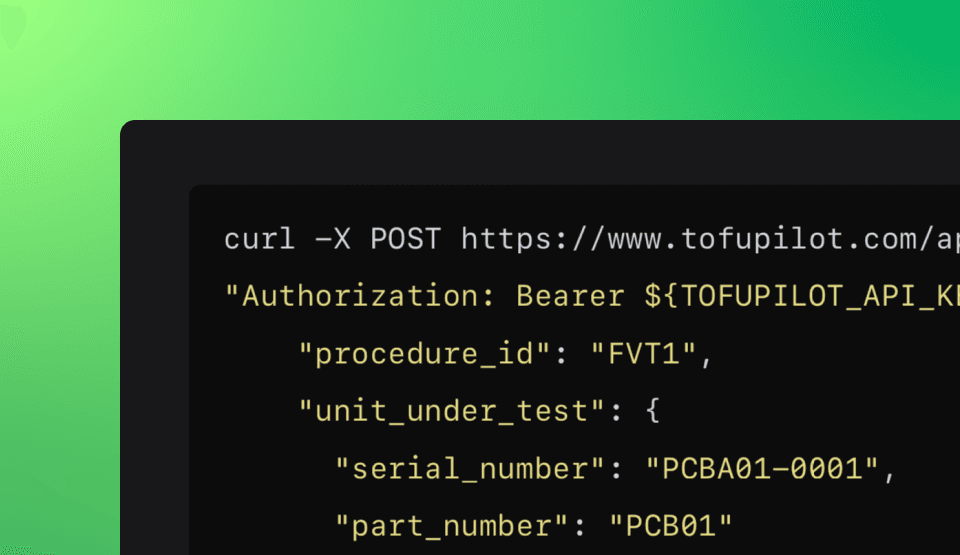
Overview
The TofuPilot REST API is the main interface for uploading test runs programmatically. It allows you to authenticate, upload test data, retrieve information about your runs, and update the unit under test.
Authentication
Authenticate your requests to access any endpoints in the TofuPilot API using OAuth2 with your API key.
Add the key to the request header using cURL:
curl https://tofupilot.app/api/v1/runs \
-H "Authorization: Bearer ${TOFUPILOT_API_KEY}"
Keep your API key safe and regenerate it if you suspect compromise.
Create a run
This endpoint allows you to create a new run.
- Name
procedure_id
- Type
- string, required
- Description
Unique identifier for the procedure. Example: "FVT1"
- Name
unit_under_test
- Type
- record, required
- Description
Record of the unit under test.
- Name
serial_number
- Type
- string, required
- Description
Unit identifier. Creates a new unit if no match. Example: "SN123456"
- Name
part_number
- Type
- string, optional
- Description
Component identifier. Creates a new component if no match. Example: "PN-001"
- Name
part_name
- Type
- string, optional
- Description
Assigned name for the component, applied when creating a new one. Example: "Custom Widget"
- Name
revision
- Type
- string, optional
- Description
Component revision. Required if multiple revisions exist. Example: "A"
- Name
run_passed
- Type
- boolean, required
- Description
Indicates if the run passed. Example: true
- Name
started_at
- Type
- string, optional
- Description
ISO 8601 start time of the run. Example: "2024-09-11T08:00:00Z"
- Name
duration
- Type
- string, optional
- Description
The duration of the run.
- For REST API: Use the ISO 8601 duration format. Example: "PT1H23M12S"
- For Python Client: Use a
timedelta
object. Example:timedelta(hours=1, minutes=23, seconds=12)
- Name
phases
- Type
- array, optional
- Description
Phases of the run.
- Name
name
- Type
- string, required
- Description
The name of the phase. Example: "temperature_calibration"
- Name
outcome
- Type
- "PASS" | "FAIL" | "ERROR" | "SKIP", required
- Description
Outcome of the phase. Example: "PASS"
- Name
start_time_millis
- Type
- number
- Description
A Unix epoch timestamp in milliseconds representing the start time of the phase. Example: 1726147200000
- Name
end_time_millis
- Type
- number
- Description
A Unix epoch timestamp in milliseconds representing the end time of the phase. Example: 1726147230000
- Name
measurements
- Type
- array, optional
- Description
The array of one or several measurement in one phase.
- Name
name
- Type
- string, required
- Description
The name of one measurement.
- Name
outcome
- Type
- "PASS" | "FAIL" | "UNSET", required
- Description
outcome of the measurement. Example: "PASS"
- Name
measured_value
- Type
- number | string | boolean | JSON, optional
- Description
The value of the measurement.
- Name
units
- Type
- string, optional
- Description
The units of the value. Examples: Celsius Degrees, Voltage
- Name
lower_limit
- Type
- number, optional
- Description
Minimum threshold. Only taken into account if measured_value is a number. Example: 3.2
- Name
upper_limit
- Type
- number, optional
- Description
Maximum threshold. Only taken into account if measured_value is a number. Example: 13.2
- Name
attachments
- Type
- array(string), optional
- Description
File paths for attachments. Example: ["path/to/file1.png", "path/to/file2.pdf"]
- Name
sub_units
- Type
- array(record), optional
- Description
Identifiers of sub-units.
- Name
serial_number
- Type
- string, required
- Description
Serial number of sub-unit. Example: "SN789"
Request
from tofupilot import TofuPilotClient
from datetime import timedelta
client = TofuPilotClient()
client.create_run(
procedure_id="FVT1",
unit_under_test={
"serial_number": 'PCBA01-0001',
"part_number": 'PCB01'
},
run_passed=True,
duration=timedelta(minutes=27, seconds=15),
)
Response
{
"message": "Run created successfully: https://tofupilot.app/yourcompany/runs/your-new-run"
}
Create a run from a file
This endpoint allows you to create a new run from a file report.
- Name
file_path
- Type
- string, required
- Description
The path to the log file to be imported.
Request
from tofupilot import TofuPilotClient
client = TofuPilotClient()
client.create_run_from_report(
file_path="path/to/your/test/report.json",)
Response
{
"message": "Run created successfully: https://tofupilot.app/yourcompany/runs/your-new-run"
}
Get runs by serial number
This endpoint allows you to fetch all runs related to a specific unit.
Request
from tofupilot import TofuPilotClient
client = TofuPilotClient()
client.get_runs(serial_number="PCBA01-0001")
Response
{
"message": "Runs fetched successfuly.",
"data": [
{
"duration": "PT12S",
"createdAt": "2024-09-05T15:58:30.891Z",
"startedAt": "2024-09-05T08:38:17.115Z",
"createdBy": {
"email": "user@acmecorp.com"
},
"status": {
"name": "Pass",
"category": "Successful"
},
"phases": [
{
"name": "measure_duration",
"startedAt": "2024-12-20T15:12:26.397Z",
"duration": "PT11S",
"outcome": "PASS",
"measurements": [
"name": "current_duration",
"measured_value": 5,
"outcome": "PASS",
"units": "second",
"lowerLimit": 1,
"upperLimit": 10
],
}
]
}
]
}
Delete a run
This endpoint allows you to delete a specific run.
The id of a run can be found by clicking its shortened version on its page.
Request
from tofupilot import TofuPilotClient
client = TofuPilotClient()
client.delete_run(
run_id="005f1ec0-b179-11ef-9a12-f7e5c5919019",
)
Response
{
"message": "Run 005f1ec0-b179-11ef-9a12-f7e5c5919019 deleted successfully."
}
Update a unit
This endpoint allows you to add sub-units to a specific unit.
- Name
sub_units
- Type
- array(record), optional
- Description
Identifiers of sub-units.
Request
from tofupilot import TofuPilotClient
client = TofuPilotClient()
client.update_unit(
serial_number="PCBA01-0001",
sub_units=[
{"serial_number": "CELL01-0001"},
{"serial_number": "CELL01-0002"},
],
)
Response
{
"message": "Unit PCBA01-0001 updated successfully."
}
Delete a unit
This endpoint allows you to delete a specific unit.
A unit can only be deleted after all its associated runs have been deleted.
Request
from tofupilot import TofuPilotClient
client = TofuPilotClient()
client.delete_unit(
serial_number="PCBA01-0001",
)
Response
{
"message": "Unit PCBA01-0001 deleted successfully."
}